File encoding is the process of converting data into a specific format that can be stored and processed by a computer. Different encoding formats are used to represent characters, numbers, and other data in a way that is understandable by software applications and operating systems. Checking the file encoding format is important because incorrect encoding can lead to issues such as garbled text, incorrect data interpretation, and compatibility problems between different systems and applications.
Method 1: Using Text Editors
Notepad++: It is a popular text editor for Windows. Open the file in Notepad++. Go to the “Encoding” menu. It will display the current encoding of the file. If the file is opened with the wrong encoding, you can try different encoding options from the menu until the text appears correctly. For example, if you have a file that contains Chinese characters and it is opened with the wrong encoding, the characters will appear as random symbols. By trying different encodings like UTF – 8 or GBK, you can find the correct one that displays the characters properly.
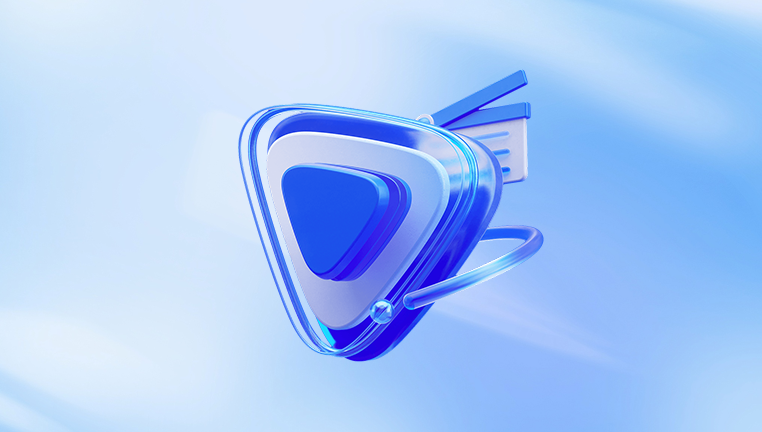
Sublime Text: Another widely used text editor. Open the file in Sublime Text. You can check the encoding in the status bar at the bottom of the window. If the encoding is not correct, you can change it by going to the “Encoding” option in the “View” menu and selecting the appropriate encoding.
Vim: In the Vim text editor, you can use the :set fileencoding? command to check the encoding of the currently opened file. To change the encoding, you can use commands like :set fileencoding=utf – 8 to set it to UTF – 8.
Method 2: Using Command – Line Tools
file command in Linux and macOS: The file command is a powerful tool in Unix – like systems. For example, if you want to check the encoding of a file named example.txt, you can run the command file -i example.txt. The output will show the MIME type and the encoding of the file. For a UTF – 8 encoded text file, it might show something like example.txt: text/plain; charset=utf – 8.
chcp command in Windows: In the Windows Command Prompt, the chcp command is used to display or change the active code page. To check the current code page (encoding) of the console, run chcp. The number displayed corresponds to a specific encoding. For example, code page 65001 represents UTF – 8. However, this method is more related to the console encoding rather than the encoding of a specific file. To check the encoding of a text file in Windows using the command line, you can use tools like type or more to view the file contents. If the text appears garbled, it may indicate that the file is not encoded in the expected format. You can then try to open the file in a text editor and check or change the encoding as described above.
Method 3: Using Programming Languages
Python: The chardet library in Python can be used to detect the encoding of a file. First, install the chardet library using pip install chardet. Then, you can use the following code to check the encoding of a file:
python
import chardet
def check_encoding(file_path):
with open(file_path, ‘rb’) as f:
data = f.read()
result = chardet.detect(data)
return result[‘encoding’]
file_path = ‘example.txt’
print(check_encoding(file_path))
Java: In Java, you can use the Charset class to detect the encoding of a file. Here is an example code:
java
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.nio.charset.Charset;
public class FileEncodingChecker {
public static void main(String[] args) {
String file = “example.txt”;
try (BufferedReader reader = new BufferedReader(new InputStreamReader(new FileInputStream(file), Charset.forName(“UTF-8”)))) {
// Try to read the file with UTF – 8 encoding
// If it fails, you can try other encodings
String line;
while ((line = reader.readLine())!= null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
C#: In C#, you can use the Encoding class to detect the encoding of a file. Here is an example:
csharp
using System;
using System.IO;
using System.Text;
class Program {
static void Main() {
string file = “example.txt”;
try {
// Try to read the file with UTF – 8 encoding
using (StreamReader reader = new StreamReader(file, Encoding.UTF8)) {
string line;
while ((line = reader.ReadLine())!= null) {
Console.WriteLine(line);
}
}
} catch (IOException e) {
Console.WriteLine(e.Message);
}
}
}
Method 4: Using File Properties in Operating Systems
Windows: Right – click on the file, select “Properties”. In the Properties window, go to the “Details” tab. Look for the “File system” section. It may show some information about the encoding if it is available. However, this information is not always accurate or detailed. For example, for a text file, it might not show the specific encoding used.
macOS: Select the file and choose “Get Info” from the “File” menu or use the shortcut Command + I. In the Info window, look for the “General” section. There is usually no direct information about the file encoding here. But for some file types, such as text files, you can open the file in a text editor and check the encoding as described earlier.
Special Cases and Considerations
Binary Files
Binary files, such as images, videos, and executables, have a different structure and encoding compared to text files. They are not encoded in the same way as text files using character encodings. For example, a JPEG image file has a specific binary format that follows the JPEG standard. To check the format of a binary file, you can use tools that are specific to that file type. For example, image editing software can usually identify the format of an image file. For video files, media players or video editing software can detect the file format and codec used.
Encrypted Files
Encrypted files pose a challenge when it comes to checking the encoding. Since the data is encrypted, the original encoding is hidden and cannot be directly accessed or determined without decrypting the file first. If you have the appropriate decryption key and software, you can decrypt the file and then check the encoding of the decrypted content. However, if you don’t have the decryption key, it is impossible to determine the original encoding.
Multilingual Files
Files that contain text in multiple languages often use encodings that can support a wide range of characters. UTF – 8 is a popular encoding for multilingual text because it can represent characters from almost all languages. When checking the encoding of a multilingual file, make sure to use tools and methods that can handle a wide range of characters. If a file contains characters from different languages and is not encoded properly, it may result in some characters being displayed incorrectly. For example, if a file contains both Chinese and English characters and is encoded in a single – byte encoding like ISO – 8859 – 1 that does not support Chinese characters, the Chinese characters will appear as garbled text.
Checking the file encoding format is an important task to ensure the proper interpretation and display of data. There are various methods available, depending on your operating system, the tools you have installed, and your programming language of choice. Text editors provide a simple and convenient way to check and change the encoding for text files. Command – line tools are useful for quick checks in Unix – like systems.
About us and this blog
Panda Assistant is built on the latest data recovery algorithms, ensuring that no file is too damaged, too lost, or too corrupted to be recovered.
Request a free quote
We believe that data recovery shouldn’t be a daunting task. That’s why we’ve designed Panda Assistant to be as easy to use as it is powerful. With a few clicks, you can initiate a scan, preview recoverable files, and restore your data all within a matter of minutes.
Subscribe to our newsletter!
More from our blog
See all postsRecent Posts
- What does unsupported file format mean? 2025-04-07
- What file format does cricut use? 2025-04-07
- What file format do 3d printers use? 2025-04-07