In the world of big data processing, Apache Spark is a popular open-source framework that has become synonymous with distributed data processing. PySpark is the Python API for Apache Spark, which allows developers to interface with Spark using the Python programming language. One of the many powerful features of PySpark is its ability to handle various file formats, including the Parquet format. Parquet is a columnar storage format that is widely used for efficient data storage and retrieval in big data environments.
The Parquet file format is designed to store large datasets in a way that is highly efficient for both storage and performance. Due to its columnar nature, Parquet files are particularly well-suited for use cases where you need to perform complex analytical queries, especially on massive datasets. PySpark’s ability to read and write Parquet files efficiently makes it a powerful tool for working with big data, where scalability, speed, and optimization are crucial.
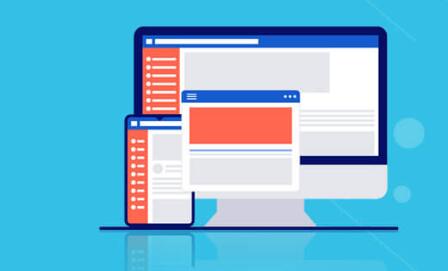
1. Installing PySpark
You can install PySpark using Python’s package manager, pip. To do this, run the following command:
bash
pip install pyspark
If you are working in a distributed environment, you may need to configure the necessary Hadoop and Spark setups. However, for local development, the pip installation will suffice.
2. Setting up a SparkSession
In PySpark, the entry point to interacting with Spark is the SparkSession. The SparkSession allows you to configure the Spark application and connect to various data sources, including Parquet files. You can create a SparkSession as follows:
python
from pyspark.sql import SparkSession # Create a SparkSession spark = SparkSession.builder \ .appName(“ParquetFileSearch”) \ .getOrCreate()
3. Verifying the PySpark Installation
To ensure that PySpark has been correctly installed, you can run a basic test to check the version of Spark:
python
print(spark.version)
This should output the version of Spark that PySpark is using, confirming that the setup is correct.
Understanding Parquet Files
Parquet is a popular columnar storage format that is optimized for both storage efficiency and query performance. The format is highly compressed and supports schema evolution, making it a great choice for large datasets in distributed computing environments.
A Parquet file is made up of the following components:
Row Groups: A Parquet file is divided into row groups, which are collections of rows of data that are stored together. Each row group contains a columnar representation of the data, enabling efficient column-based queries.
Column Chunks: Within each row group, the data for each column is stored in separate chunks. These chunks are compressed, further optimizing the storage and performance of the data.
Metadata: The file includes metadata that describes the structure and schema of the data, such as the types of columns, the number of rows, and the compression algorithm used.
PySpark’s ability to efficiently read and write Parquet files comes from its underlying use of Hadoop’s Parquet library, which ensures that the files are processed in a distributed manner, enabling scalability and performance.
Finding Parquet Files in Your File System
In many big data use cases, Parquet files are distributed across multiple directories or stored in cloud-based storage systems like Amazon S3. Google Cloud Storage, or Azure Blob Storage. To locate Parquet files in your environment, you can use PySpark’s file system interface.
1. Searching for Files in Local Directories
If you are working with local directories, you can use Python’s built-in libraries like os or glob to search for Parquet files. However, PySpark offers a more distributed and scalable way to locate files using the SparkContext and its ability to interact with Hadoop’s distributed file system (HDFS).
python
import os # Define the directory where Parquet files are located directory_path = “/path/to/your/data/” # Search for all Parquet files in the directory parquet_files = [f for f in os.listdir(directory_path) if f.endswith(‘.parquet’)] # Print the list of found Parquet files print(parquet_files)
This will give you a list of all files with the .parquet extension in the specified directory.
2. Searching Parquet Files in HDFS or Cloud Storage
In a distributed environment, your data is likely stored in HDFS or a cloud storage system. PySpark can connect to these systems, allowing you to search for Parquet files in these storage solutions. Here’s an example of how you can list all Parquet files in an HDFS directory:
python
# List files in HDFS parquet_files_hdfs = spark._jvm.org.apache.hadoop.fs.FileSystem \ .get(spark._jsc.hadoopConfiguration()) \ .listStatus(spark._jvm.org.apache.hadoop.fs.Path(“/path/to/hdfs/”)) # Filter and display only Parquet files parquet_files_hdfs = [f.getPath().toString() for f in parquet_files_hdfs if f.getPath().toString().endswith(‘.parquet’)] print(parquet_files_hdfs)
Similarly, if you’re working with cloud storage, PySpark can interface with systems like Amazon S3. Google Cloud Storage, or Azure Blob Storage by using the appropriate Hadoop configurations.
Reading Parquet Files with PySpark
Once you’ve located the Parquet files, the next step is to read them into a PySpark DataFrame for processing. PySpark provides a simple interface for reading Parquet files through the read.parquet() method.
1. Reading a Single Parquet File
To read a single Parquet file, simply provide the path to the file as an argument:
python
# Read a single Parquet file into a DataFrame df = spark.read.parquet(“/path/to/your/file.parquet”) # Show the first few rows of the DataFrame df.show()
2. Reading Multiple Parquet Files
You can also read multiple Parquet files at once by providing a directory or a list of file paths. PySpark will automatically read all the files in the specified location.
python
# Read multiple Parquet files from a directory df_multiple_files = spark.read.parquet(“/path/to/your/files/*.parquet”) # Show the first few rows of the DataFrame df_multiple_files.show()
This flexibility allows you to load entire datasets, even if they are spread across multiple files.
3. Reading Parquet Files from Cloud Storage
If you’re working with cloud storage, PySpark can read Parquet files directly from services like Amazon S3. To read Parquet files from S3. you need to specify the S3 path and ensure the proper credentials are configured.
python
# Read Parquet files from Amazon S3 df_s3 = spark.read.parquet(“s3a://your-bucket-name/path/to/files/*.parquet”) # Show the first few rows of the DataFrame df_s3.show()
You must configure the necessary credentials for accessing the cloud storage, which can be done through environment variables, AWS credentials files, or by providing the credentials directly in your Spark session configuration.
Optimizing Parquet File Handling
When working with large datasets stored in Parquet files, performance optimization becomes crucial. Here are a few tips for optimizing your use of Parquet files in PySpark:
1. Partitioning Parquet Files
Partitioning your data into smaller, manageable pieces based on certain column values can greatly improve query performance. When reading Parquet files, PySpark will only read the necessary partitions, reducing the amount of data that needs to be processed.
python
# Save a DataFrame as a partitioned Parquet file df.write.partitionBy(“column_name”).parquet(“/path/to/output/partitioned_parquet”)
2. Column Pruning
One of the biggest advantages of Parquet files is their columnar format, which allows you to read only the columns you need. PySpark allows you to specify which columns to load when reading Parquet files, improving performance by not loading unnecessary data.
python
# Read only specific columns from a Parquet file df_selected_columns = spark.read.parquet(“/path/to/file.parquet”).select(“column1”, “column2”) df_selected_columns.show()
3. Caching DataFrames
If you need to perform multiple operations on the same dataset, caching can help speed up processing by storing the data in memory.
python
# Cache the DataFrame in memory df.cache() # Perform operations on the cached DataFrame df.show()
PySpark is a powerful tool for processing large datasets, and its ability to work with Parquet files makes it even more valuable in big data environments. Whether you’re searching for Parquet files, reading them into a DataFrame, or optimizing your queries, PySpark offers the tools you need to efficiently work with large datasets.
About us and this blog
Panda Assistant is built on the latest data recovery algorithms, ensuring that no file is too damaged, too lost, or too corrupted to be recovered.
Request a free quote
We believe that data recovery shouldn’t be a daunting task. That’s why we’ve designed Panda Assistant to be as easy to use as it is powerful. With a few clicks, you can initiate a scan, preview recoverable files, and restore your data all within a matter of minutes.
Subscribe to our newsletter!
More from our blog
See all postsRecent Posts
- Can not open jgp files learnosity 2025-02-24
- Klipper unable to open file 2025-02-24
- File format or file extension is not valid 2025-02-24